This guide covers building a list-selection pop-up for multiple wishlists.
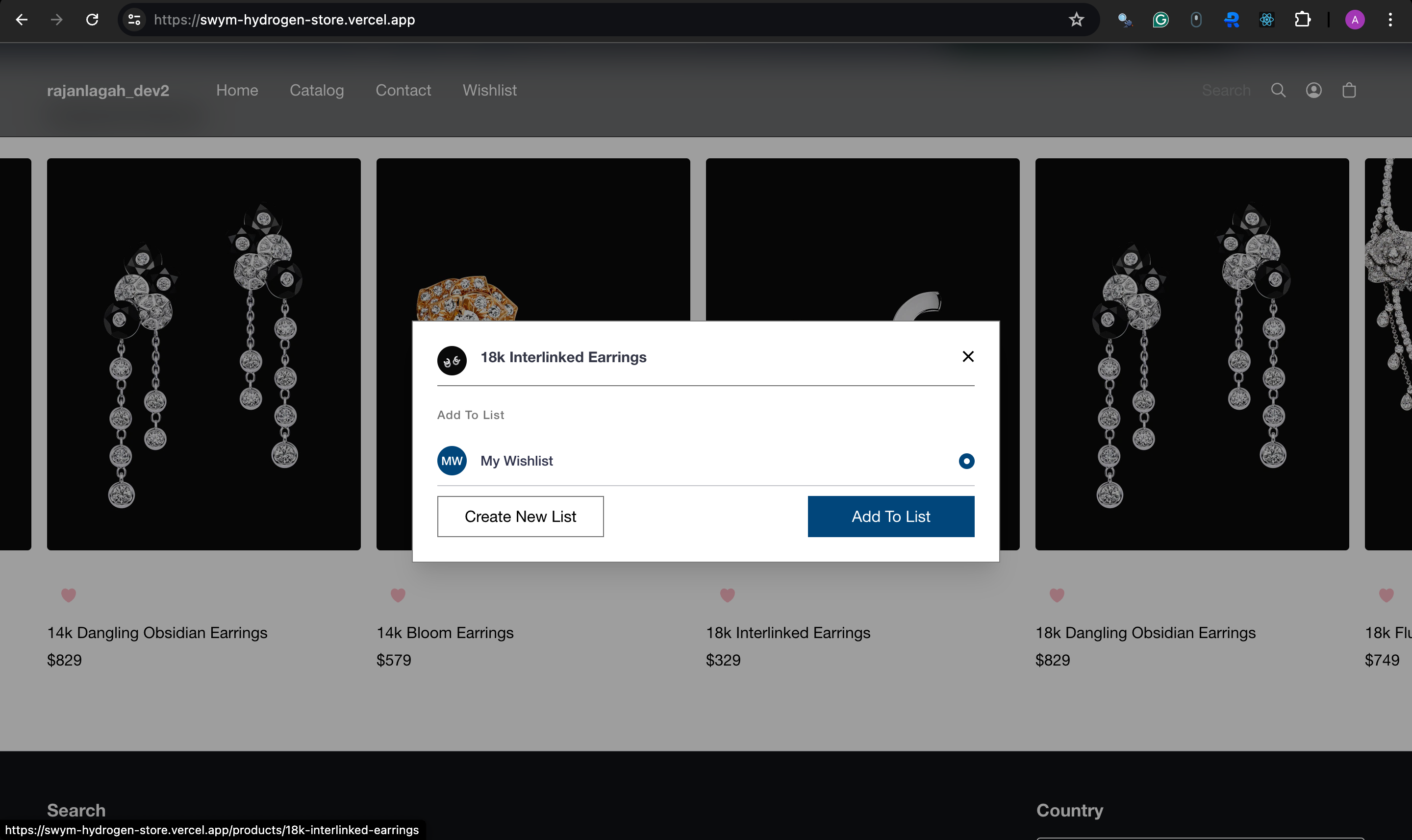
Scroll to the bottom of this page for an easy copy paste solution!
Step 1: Import APIs and Components
Code Overview:
createList, fetchList, AddToWishlist : These can be found in the Swym API server file shared in the second page of this guide. In case you haven't added them, add them )[from this link.](https://developers.swym.it/reference/pepare-client-apis
WishlistNameItem, DataContext: These can be found in the Swym components folder shared in the first page of this guide. In case you haven't added them, add them )[from this link.](https://github.com/swym-corp/swym-hydrogen-store/tree/headless-ui-update/src/components/wishlist
setSwymLocalStorageListData: These can be found in the Swym utils file shared in the first page of this guide. In case you haven't added them, add them from this link.
SWYM_CONFIG: This file needs to be setup to store API keys, endpoints, etc. If you haven't set it up already, click here.
Import components like WishlistNameItem and DataContext.
import { useContext, useEffect, useState } from 'react';
import './addToListPopup.css';
import WishlistNameItem from './WishlistNameItem.client';
import { DataContext } from '../wishlist-context';
import { AddToWishlist, createList, fetchList } from '../../../swym/api/store-apis';
import { setSwymLocalStorageListData } from '../../../swym/Utils/Utils';
import SWYM_CONFIG from '../../../swym/swym.config';
Step 2: Define Helper Functions
Code Overview:
Define functions to Get and Set wishlist data, Create new lists, Validate list names, and Add products to the wishlist.
const getSetList = async () => {
try {
setshowListLoading(true);
const res = await fetchList();
setSwymLocalStorageListData(res);
setshowListLoading(false);
if (res && res.length > 0) {
setsavedList(res);
setselectedCustomNameIndex(0);
}else{
setshowCreateNewList(true);
}
} catch (e) {
console.error('Exception', e);
}
};
const createNewList = () => {
setshowCreateNewList(true);
}
const hideCreateNewList = () => {
setshowCreateNewList(false);
}
const createListByName = async () => {
if (!error) {
try {
setshowLoading(true);
const res = await createList(wishlistName);
setshowLoading(false);
if (res.di) {
setsavedList([...savedList, res]);
setshowAlertBox(true);
setalertBox({ type:'success', title: 'success', info: 'List Created', image });
setWishlistName('');
hideCreateNewList();
return res;
} else {
setshowAlertBox(true);
setalertBox({ type:'error', title: 'Error', info: 'List not created', image });
}
} catch (e) {
console.error('Exception', e);
setshowAlertBox(true);
setalertBox({ type:'error', title: 'Error', info: 'List not created', image });
}
} else {
setshowAlertBox(true);
setalertBox({ type:'error', title: 'Failed', info: 'List not created', image });
setWishlistName('');
}
};
function validateWishlistName(name) {
let wishlists = [];
{
savedList &&
savedList.length > 0 &&
savedList.map((list) => {
wishlists.push(list);
});
}
let validateStringVar = validateString(name, {
empty: 'Must provide a list name',
minLength: 'Name must be longer than 3 characters',
maxLength: 'Name must be less than 50 characters long',
});
let validateUniqueStringVar = validateUniqueString(
name,
wishlists.map((list) => {
return list.lname;
}),
'List name already exists',
);
return validateStringVar || validateUniqueStringVar;
}
function validateAndSetListName(value) {
setWishlistName(value);
setError(validateWishlistName(value));
console.log(error);
}
const addProductToWishlist = async () => {
try {
let selectedListName = savedList[selectedCustomNameIndex]?.lid;
if (wishlistName && wishlistName.length > 0) {
let newList = await createListByName();
selectedListName = newList.lid;
}
setshowLoading(true);
let res = await AddToWishlist(
productId,
variantId,
productUrl,
selectedListName,
);
setshowLoading(false);
if (res?.a) {
setshowAlertBox(true);
setalertBox({ type:'success', title: 'success', info:'Product addded to wishlist', image });
onPopupToggle(false);
getSetList();
onAddedToWishlist && onAddedToWishlist();
} else {
setshowAlertBox(true);
setalertBox({ type:'error', title: 'Error', info: 'Product not added to wishlist', image });
}
} catch (e) {
console.log('Exception', e);
setshowLoading(false);
setshowAlertBox(true);
setalertBox({ type:'error', title: 'Error', info: 'Product not added to wishlist', image });
}
};
export function validateString(name, errorsObj) {
if (!name) {
return errorsObj.empty;
}
if (name.length < 3) {
return errorsObj.minLength;
}
if (name.length > 50) {
return errorsObj.maxLength;
}
}
export const validateUniqueString = (newList, previousListArr, errorStr) => {
if (previousListArr.indexOf(newList) !== -1) {
return errorStr;
}
};
Step 3: Create a Functional Component Loader to show a loading spinner.
Code Overview:
Create a functional component Loader to show a loading spinner.
function Loader({showLoading, width = 30 }){
return (
<>
{showLoading &&
<div className='swym-hl-modal-loader'>
<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 200 200" width={width}><radialGradient id="a12" cx=".66" fx=".66" cy=".3125" fy=".3125" gradientTransform="scale(1.5)"><stop offset="0" stopColor="currentColor"></stop><stop offset=".3" stopColor="currentColor" stopOpacity=".9"></stop><stop offset=".6" stopColor="currentColor" stopOpacity=".6"></stop><stop offset=".8" stopColor="currentColor" stopOpacity=".3"></stop><stop offset="1" stopColor="currentColor" stopOpacity="0"></stop></radialGradient><circle transform-origin="center" fill="none" stroke="url(#a12)" strokeWidth="15" strokeLinecap="round" strokeDasharray="200 1000" strokeDashoffset="0" cx="100" cy="100" r="70"><animateTransform type="rotate" attributeName="transform" calcMode="spline" dur="2" values="360;0" keyTimes="0;1" keySplines="0 0 1 1" repeatCount="indefinite"></animateTransform></circle><circle transform-origin="center" fill="none" opacity=".2" stroke="currentColor" strokeWidth="15" strokeLinecap="round" cx="100" cy="100" r="70"></circle></svg>
</div>
}
</>
)
}
Step 4: Define the AddToListPopup Component and Initialize State Variables
Code Overview:
Create a functional component AddToListPopup to handle adding products to a list and creating new lists.
Use useState to manage the necessary states.
Use useEffect to fetch and set the wishlist data on component mount.
export default function AddToListPopup({title, productId, variantId, productUrl, image, onPopupToggle, setshowAlertBox, setalertBox, onAddedToWishlist}){
const [showCreateNewList, setshowCreateNewList] = useState(false);
const [showLoading, setshowLoading] = useState(false);
const [showListLoading, setshowListLoading] = useState(false);
const [customListName, setcustomListName] = useState('');
const [wishlistName, setWishlistName] = useState('');
const [error, setError] = useState();
const {
selectedCustomNameIndex,
setselectedCustomNameIndex,
savedList,
setsavedList,
WishlistText,
setWishlistText,
} = useContext(DataContext);
useEffect(() => {
getSetList();
}, []);
Step 5: Render the Component
Code Overview:
Return the JSX structure which includes the pop-up structure, input for creating new lists, and the list of existing wishlists.
return (
<div id="swym-hl-add-to-list-popup" className="modal" onClick={(e)=> { e.preventDefault(); e.stopPropagation(); onPopupToggle(false) }}>
<div className="swym-hl-modal-content" onClick={(e)=>{e.preventDefault(); e.stopPropagation();}}>
<span className="swym-hl-modal-close-btn" onClick={()=>onPopupToggle(false)}>×</span>
<div className="swym-hl-product-title">
<div className="swym-hl-product-image">
<img src={image} alt="" />
</div>
<h3 className="swym-product-name swym-heading swym-heading-1 swym-title-new">{title}</h3>
</div>
<div className="swym-hl-product-content">
{showCreateNewList && (
<div>
<div className="swym-hl-new-wishlist-item swym-hl-new-wishlist-input-container">
<input
type="text"
className={`swym-new-wishlist-name swym-no-zoom-fix swym-input ${error ? 'swym-input-has-error' : ''}`}
onChange={(e) => {
validateAndSetListName(e.target.value);
}}
placeholder={SWYM_CONFIG.defaultWishlistName}
value={wishlistName}
/>
<span className="error-msg" role="alert">
{error}
</span>
</div>
<div className='swym-hl-modal-action'>
<button className='swym-hl-bg-outline swym-hl-modal-action-btn' onClick={hideCreateNewList} >cancel</button>
<button className='swym-hl-bg-color swym-hl-modal-action-btn swym-hl-text-color' onClick={(e)=>{ e.preventDefault(); e.stopPropagation(); createListByName()}}> <Loader width={20} showLoading={showLoading} /> Create</button>
</div>
</div>
)}
{!showCreateNewList && (
<div>
<div className="swym-wishlist-items">
<div className="swym-wishlist-items-title" role="radiogroup">Add To List</div>
{ ( !savedList || !savedList.length ) && <Loader showLoading={showListLoading} /> }
{savedList &&
savedList.length > 0 &&
savedList.map(({lname, lid}, index) => {
return (
<WishlistNameItem
key={lid}
name={lname}
id={lid}
index={index}
/>
);
})}
</div>
<div className='swym-hl-modal-action'>
<button className='swym-hl-bg-outline swym-hl-modal-action-btn' onClick={createNewList} >Create New List</button>
<button className='swym-hl-bg-color swym-hl-modal-action-btn swym-hl-text-color' onClick={()=>addProductToWishlist()}> <Loader width={20} showLoading={showLoading} /> Add To List</button>
</div>
</div>
)}
</div>
</div>
</div>
)
.swym-bg-2 {
background: #035587 !important;
}
.swym-color-4 {
color: white;
}
#swym-hl-add-to-list-popup{
position: fixed;
top: 0;
right: 0;
bottom: 0;
left: 0;
z-index: 10000;
display: flex;
justify-content: center;
align-items: center;
width: 100vw;
height: 100vh;
background-color: #00000061;
}
.swym-hl-modal-content {
margin: auto;
padding: 20px;
border: 1px solid #888;
display: flex;
flex-direction: column;
color: #000000;
background-color: #ffffff;
box-shadow: 0 20px 25px -5px rgba(0, 0, 0, 0.1), 0 10px 10px -5px rgba(0, 0, 0, 0.04);
width: 600px;
position: relative;
}
.swym-hl-modal-close-btn{
padding: 4px;
position: absolute;
top: 5px;
right: 15px;
font-size: 25px;
z-index: 100;
cursor: pointer;
}
.swym-hl-product-title {
display: flex;
position: relative;
box-sizing: border-box;
border-bottom: 1px solid #828282;
padding-left: 0px;
padding-top: 0px;
padding-bottom: 10px;
}
.swym-hl-product-image {
width: 30px;
height: 30px;
border-radius: 30px;
display: inline-block;
margin-right: 14px;
overflow: hidden;
flex-shrink: 0;
}
.swym-hl-modal-loader{
display: flex;
justify-content: center;
align-item: center;
margin-right: 5px;
}
.swym-hl-modal-action-btn{
cursor: pointer;
padding: 8px 26px;
margin-top: 10px;
display: flex;
align-items: center;
justify-content: center;
min-width: 170px;
}
.swym-hl-modal-action {
display: flex;
justify-content: space-between;
flex-wrap: wrap;
}
.swym-hl-new-wishlist-input-container{
display: flex;
flex-direction: column;
align-items: flex-start;
padding-right: 0px;
margin-top: 20px;
}
.swym-hl-new-wishlist-item .swym-new-wishlist-name {
background: #fff;
flex-grow: 1;
font-weight: 500;
font-size: 14px;
line-height: 14px;
color: #434655;
border: 1px solid #bababa;
letter-spacing: 0.05em;
padding: 12px 14px;
width: 100%;
}
.swym-hl-new-wishlist-item .swym-new-wishlist-name:focus {
border: 1px solid #dc3b1a;
padding: 12px 14px;
}
.swym-hl-new-wishlist-item .error-msg {
display: block;
padding: 5px;
}
.swym-hl-new-wishlist-item .swym-icon {
position: absolute;
top: 24px;
right: 0;
}
.swym-hl-new-wishlist-item .swym-icon::before {
content: "\e908";
font-size: 15px;
}
.swym-hl-new-wishlist-item.swym-selected .swym-icon::before {
content: "\e907";
}
.swym-hl-new-wishlist-item:last-child {
border: 0;
}
@media screen and (max-width: 450px) {
.swym-hl-modal-action-btn{
width: 100%;
}
}
.error-msg {
font-size: 12px;
line-height: 14px;
letter-spacing: 0.05em;
color: #CC0000;
font-style: italic;
padding: 5px;
}
.swym-product-name {
line-height: 30px;
color: #434655;
font-weight: bold;
font-size: 18px;
}
.swym-heading, .swym-label {
font-size: 100%;
font: inherit;
font-family: "HelveticaNeue", Helvetica, Verdana, Arial, sans-serif;
line-height: 1.4em;
vertical-align: baseline;
box-sizing: border-box;
border: 0;
-moz-box-sizing: border-box;
-ms-box-sizing: border-box;
-webkit-box-sizing: border-box;
margin: 0;
padding: 0;
text-transform: none;
text-align: left;
}
.swym-heading-1 {
font-weight: bold;
font-size: 15px;
line-height: 22px;
color: #434655;
}
One Step Copy Paste!
import { useContext, useEffect, useState } from 'react';
import './addToListPopup.css';
import WishlistNameItem from './WishlistNameItem.client';
import { DataContext } from '../wishlist-context';
import { AddToWishlist, createList, fetchList } from '../../../swym/api/store-apis';
import { setSwymLocalStorageListData } from '../../../swym/Utils/Utils';
import SWYM_CONFIG from '../../../swym/swym.config';
export function validateString(name, errorsObj) {
if (!name) {
return errorsObj.empty;
}
if (name.length < 3) {
return errorsObj.minLength;
}
if (name.length > 50) {
return errorsObj.maxLength;
}
}
export const validateUniqueString = (newList, previousListArr, errorStr) => {
if (previousListArr.indexOf(newList) !== -1) {
return errorStr;
}
};
function Loader({showLoading, width = 30 }){
return (
<>
{showLoading &&
<div className='swym-hl-modal-loader'>
<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 200 200" width={width}><radialGradient id="a12" cx=".66" fx=".66" cy=".3125" fy=".3125" gradientTransform="scale(1.5)"><stop offset="0" stopColor="currentColor"></stop><stop offset=".3" stopColor="currentColor" stopOpacity=".9"></stop><stop offset=".6" stopColor="currentColor" stopOpacity=".6"></stop><stop offset=".8" stopColor="currentColor" stopOpacity=".3"></stop><stop offset="1" stopColor="currentColor" stopOpacity="0"></stop></radialGradient><circle transform-origin="center" fill="none" stroke="url(#a12)" strokeWidth="15" strokeLinecap="round" strokeDasharray="200 1000" strokeDashoffset="0" cx="100" cy="100" r="70"><animateTransform type="rotate" attributeName="transform" calcMode="spline" dur="2" values="360;0" keyTimes="0;1" keySplines="0 0 1 1" repeatCount="indefinite"></animateTransform></circle><circle transform-origin="center" fill="none" opacity=".2" stroke="currentColor" strokeWidth="15" strokeLinecap="round" cx="100" cy="100" r="70"></circle></svg>
</div>
}
</>
)
}
export default function AddToListPopup({title, productId, variantId, productUrl, image, onPopupToggle, setshowAlertBox, setalertBox, onAddedToWishlist}){
const [showCreateNewList, setshowCreateNewList] = useState(false);
const [showLoading, setshowLoading] = useState(false);
const [showListLoading, setshowListLoading] = useState(false);
const [customListName, setcustomListName] = useState('');
const [wishlistName, setWishlistName] = useState('');
const [error, setError] = useState();
const {
selectedCustomNameIndex,
setselectedCustomNameIndex,
savedList,
setsavedList,
WishlistText,
setWishlistText,
} = useContext(DataContext);
useEffect(() => {
getSetList();
}, []);
const getSetList = async () => {
try {
setshowListLoading(true);
const res = await fetchList();
setSwymLocalStorageListData(res);
setshowListLoading(false);
if (res && res.length > 0) {
setsavedList(res);
setselectedCustomNameIndex(0);
}else{
setshowCreateNewList(true);
}
console.log('this is', res);
} catch (e) {
console.log('Exception', e);
}
};
const createNewList = () => {
setshowCreateNewList(true);
}
const hideCreateNewList = () => {
setshowCreateNewList(false);
}
const createListByName = async () => {
if (!error) {
try {
setshowLoading(true);
const res = await createList(wishlistName);
setshowLoading(false);
if (res.di) {
setsavedList([...savedList, res]);
setshowAlertBox(true);
setalertBox({ type:'success', title: 'success', info: 'List Created', image });
setWishlistName('');
hideCreateNewList();
return res;
} else {
setshowAlertBox(true);
setalertBox({ type:'error', title: 'Error', info: 'List not created', image });
}
} catch (e) {
console.log('Exception', e);
setshowAlertBox(true);
setalertBox({ type:'error', title: 'Error', info: 'List not created', image });
}
} else {
setshowAlertBox(true);
setalertBox({ type:'error', title: 'Failed', info: 'List not created', image });
setWishlistName('');
}
};
function validateWishlistName(name) {
let wishlists = [];
{
savedList &&
savedList.length > 0 &&
savedList.map((list) => {
wishlists.push(list);
});
}
let validateStringVar = validateString(name, {
empty: 'Must provide a list name',
minLength: 'Name must be longer than 3 characters',
maxLength: 'Name must be less than 50 characters long',
});
let validateUniqueStringVar = validateUniqueString(
name,
wishlists.map((list) => {
return list.lname;
}),
'List name already exists',
);
return validateStringVar || validateUniqueStringVar;
}
function validateAndSetListName(value) {
setWishlistName(value);
setError(validateWishlistName(value));
console.log(error);
}
const addProductToWishlist = async () => {
try {
let selectedListName = savedList[selectedCustomNameIndex]?.lid;
if (wishlistName && wishlistName.length > 0) {
let newList = await createListByName();
selectedListName = newList.lid;
}
setshowLoading(true);
let res = await AddToWishlist(
productId,
variantId,
productUrl,
selectedListName,
);
setshowLoading(false);
if (res?.a) {
setshowAlertBox(true);
setalertBox({ type:'success', title: 'success', info:'Product addded to wishlist', image });
onPopupToggle(false);
getSetList();
onAddedToWishlist && onAddedToWishlist();
} else {
setshowAlertBox(true);
setalertBox({ type:'error', title: 'Error', info: 'Product not added to wishlist', image });
}
} catch (e) {
console.log('Exception', e);
setshowLoading(false);
setshowAlertBox(true);
setalertBox({ type:'error', title: 'Error', info: 'Product not added to wishlist', image });
}
};
return (
<div id="swym-hl-add-to-list-popup" className="modal" onClick={(e)=> { e.preventDefault(); e.stopPropagation(); onPopupToggle(false) }}>
<div className="swym-hl-modal-content" onClick={(e)=>{e.preventDefault(); e.stopPropagation();}}>
<span className="swym-hl-modal-close-btn" onClick={()=>onPopupToggle(false)}>×</span>
<div className="swym-hl-product-title">
<div className="swym-hl-product-image">
<img src={image} alt="" />
</div>
<h3 className="swym-product-name swym-heading swym-heading-1 swym-title-new">{title}</h3>
</div>
<div className="swym-hl-product-content">
{showCreateNewList && (
<div>
<div className="swym-hl-new-wishlist-item swym-hl-new-wishlist-input-container">
<input
type="text"
className={`swym-new-wishlist-name swym-no-zoom-fix swym-input ${error?'swym-input-has-error':''}`}
onChange={(e) => {
validateAndSetListName(e.target.value);
}}
placeholder={SWYM_CONFIG.defaultWishlistName}
value={wishlistName}
/>
<span className="error-msg" role="alert">
{error}
</span>
</div>
<div className='swym-hl-modal-action'>
<button className='swym-hl-bg-outline swym-hl-modal-action-btn' onClick={hideCreateNewList} >cancel</button>
<button className='swym-hl-bg-color swym-hl-modal-action-btn swym-hl-text-color' onClick={(e)=>{ e.preventDefault(); e.stopPropagation(); createListByName()}}> <Loader width={20} showLoading={showLoading} /> Create</button>
</div>
</div>
)}
{!showCreateNewList && (
<div>
<div className="swym-wishlist-items">
<div className="swym-wishlist-items-title" role="radiogroup">Add To List</div>
{ ( !savedList || !savedList.length ) && <Loader showLoading={showListLoading} /> }
{savedList &&
savedList.length > 0 &&
savedList.map(({lname, lid}, index) => {
return (
<WishlistNameItem
key={lid}
name={lname}
id={lid}
index={index}
/>
);
})}
</div>
<div className='swym-hl-modal-action'>
<button className='swym-hl-bg-outline swym-hl-modal-action-btn' onClick={createNewList} >Create New List</button>
<button className='swym-hl-bg-color swym-hl-modal-action-btn swym-hl-text-color' onClick={()=>addProductToWishlist()}> <Loader width={20} showLoading={showLoading} /> Add To List</button>
</div>
</div>
)}
</div>
</div>
</div>
)
}
.swym-bg-2 {
background: #035587 !important;
}
.swym-color-4 {
color: white;
}
#swym-hl-add-to-list-popup{
position: fixed;
top: 0;
right: 0;
bottom: 0;
left: 0;
z-index: 10000;
display: flex;
justify-content: center;
align-items: center;
width: 100vw;
height: 100vh;
background-color: #00000061;
}
.swym-hl-modal-content {
margin: auto;
padding: 20px;
border: 1px solid #888;
display: flex;
flex-direction: column;
color: #000000;
background-color: #ffffff;
box-shadow: 0 20px 25px -5px rgba(0, 0, 0, 0.1), 0 10px 10px -5px rgba(0, 0, 0, 0.04);
width: 600px;
position: relative;
}
.swym-hl-modal-close-btn{
padding: 4px;
position: absolute;
top: 5px;
right: 15px;
font-size: 25px;
z-index: 100;
cursor: pointer;
}
.swym-hl-product-title {
display: flex;
position: relative;
box-sizing: border-box;
border-bottom: 1px solid #828282;
padding-left: 0px;
padding-top: 0px;
padding-bottom: 10px;
}
.swym-hl-product-image {
width: 30px;
height: 30px;
border-radius: 30px;
display: inline-block;
margin-right: 14px;
overflow: hidden;
flex-shrink: 0;
}
.swym-hl-modal-loader{
display: flex;
justify-content: center;
align-item: center;
margin-right: 5px;
}
.swym-hl-modal-action-btn{
cursor: pointer;
padding: 8px 26px;
margin-top: 10px;
display: flex;
align-items: center;
justify-content: center;
min-width: 170px;
}
.swym-hl-modal-action {
display: flex;
justify-content: space-between;
flex-wrap: wrap;
}
.swym-hl-new-wishlist-input-container{
display: flex;
flex-direction: column;
align-items: flex-start;
padding-right: 0px;
margin-top: 20px;
}
.swym-hl-new-wishlist-item .swym-new-wishlist-name {
background: #fff;
flex-grow: 1;
font-weight: 500;
font-size: 14px;
line-height: 14px;
color: #434655;
border: 1px solid #bababa;
letter-spacing: 0.05em;
padding: 12px 14px;
width: 100%;
}
.swym-hl-new-wishlist-item .swym-new-wishlist-name:focus {
border: 1px solid #dc3b1a;
padding: 12px 14px;
}
.swym-hl-new-wishlist-item .error-msg {
display: block;
padding: 5px;
}
.swym-hl-new-wishlist-item .swym-icon {
position: absolute;
top: 24px;
right: 0;
}
.swym-hl-new-wishlist-item .swym-icon::before {
content: "\e908";
font-size: 15px;
}
.swym-hl-new-wishlist-item.swym-selected .swym-icon::before {
content: "\e907";
}
.swym-hl-new-wishlist-item:last-child {
border: 0;
}
@media screen and (max-width: 450px) {
.swym-hl-modal-action-btn{
width: 100%;
}
}
.error-msg {
font-size: 12px;
line-height: 14px;
letter-spacing: 0.05em;
color: #CC0000;
font-style: italic;
padding: 5px;
}
.swym-product-name {
line-height: 30px;
color: #434655;
font-weight: bold;
font-size: 18px;
}
.swym-heading, .swym-label {
font-size: 100%;
font: inherit;
font-family: "HelveticaNeue", Helvetica, Verdana, Arial, sans-serif;
line-height: 1.4em;
vertical-align: baseline;
box-sizing: border-box;
border: 0;
-moz-box-sizing: border-box;
-ms-box-sizing: border-box;
-webkit-box-sizing: border-box;
margin: 0;
padding: 0;
text-transform: none;
text-align: left;
}
.swym-heading-1 {
font-weight: bold;
font-size: 15px;
line-height: 22px;
color: #434655;
}