Toast Notifications
Trigger Default Swym Toast notifications
Utilize the API to trigger Success/Error toast notifications when building a custom wishlist experience with our JS APIs. Please note that JS APIs do not automatically trigger toast notifications. Using the notification API is optional, and you are welcome to use any notification engine if our notifications do not meet your requirements.
Success Notification
let successMessage = "Testing Swym Success Notification Toast";
swat.ui.showSuccessNotification({ message: successMessage });
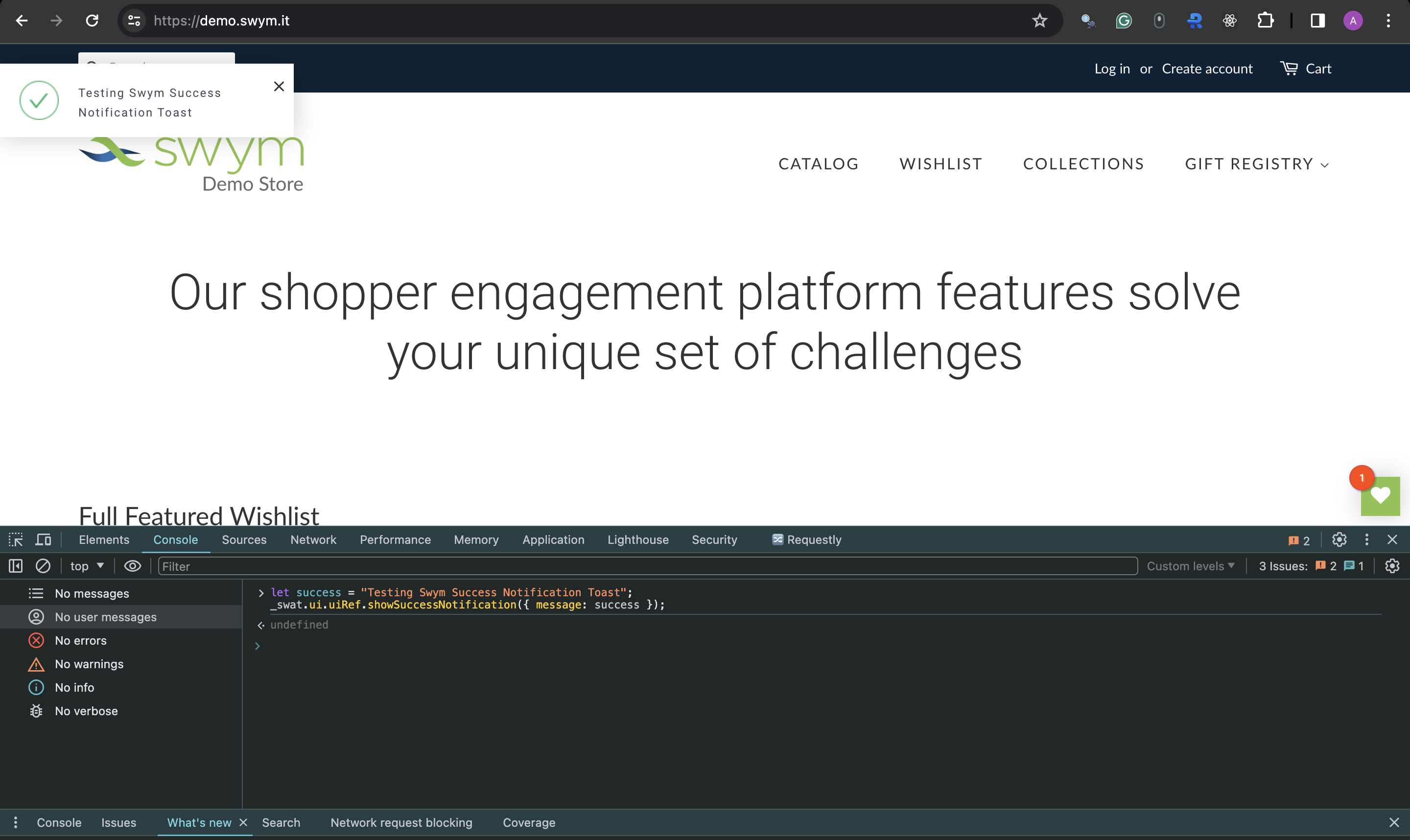
Example
/** Swym has triggered events that can be latched onto.
In this example we're latching onto the add to wishlist event layer.
**/
swat.evtLayer.addEventListener(swat.JSEvents.addedToWishlist, function(data){
// Execute code after an add to wishlist action
console.log("Add to wishlist event data:", data);
// Fetch product title name from the event data
let productTitle = data.detail.d.dt;
let successMessage = `${productTitle} has been added to wishlist!`;
// Trigger Swym success notification toast
swat.ui.showSuccessNotification({ message: successMessage });
});
Error Notification
let errorMessage = "Testing Swym Error Notification Toast";
swat.ui.showErrorNotification({ message: errorMessage });
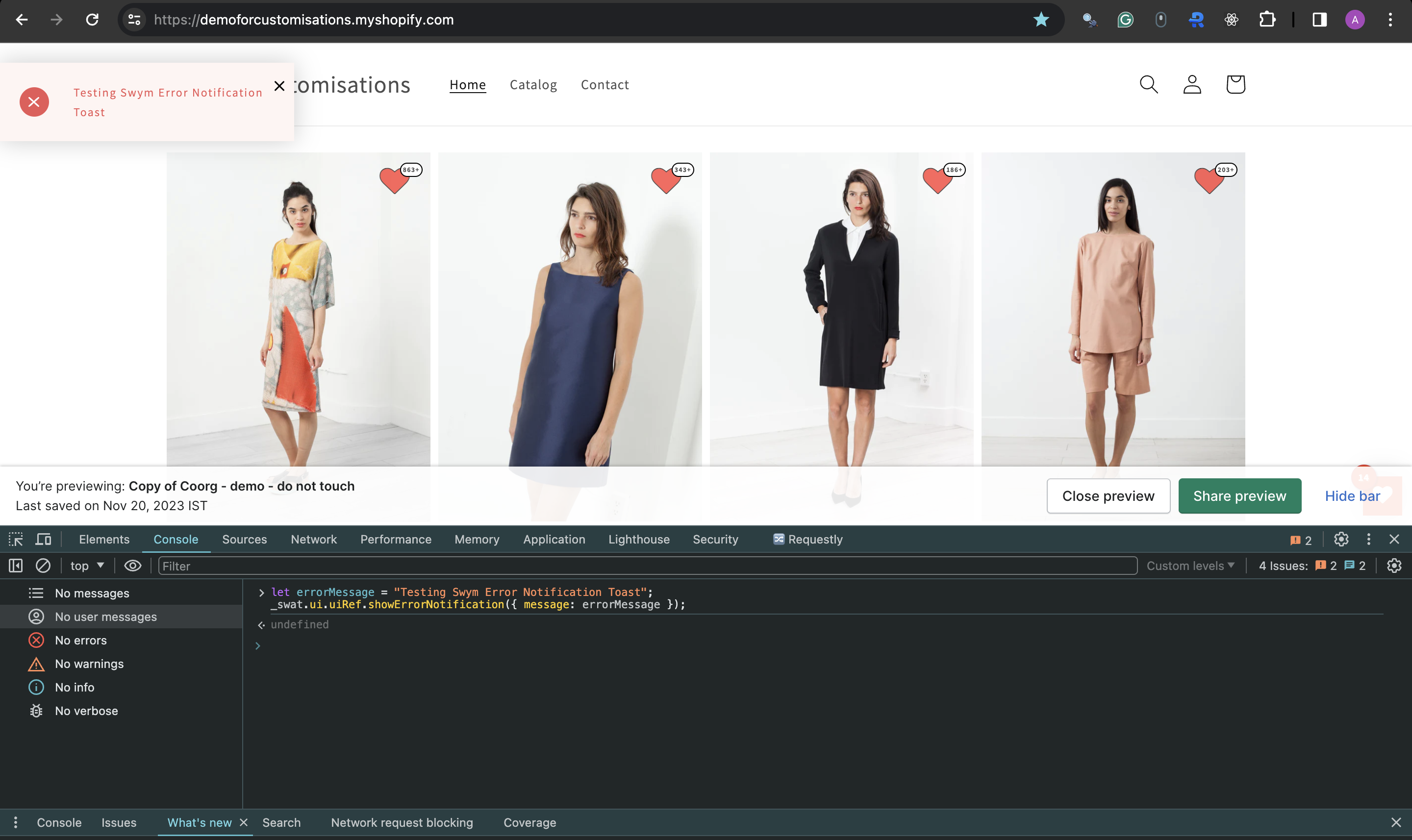
Example
In this example we're using Swym's add to cart API to Add to cart with success and error notifications
swat.replayAddToCart(
{ empi: empi, du: du, qty: qty},epi,
(success) => {
const variant = JSON.parse(success).items[0];
const productTitle = variant.title;
let successMessage = `<strong>${productTitle}</strong> has been added to cart!`;
// Show success notifcation with error description on sucessful add to cart
swat.ui.showSuccessNotification({ message:successMessage });
},
(error) => {
// Show error notifcation with error description on unsucessful add to cart
swat.ui.showErrorNotification({ message: error.description });
}
);
});
Updated 7 months ago