Create a List
Create a list or Duplicate existing lists.
swat.createList
Creates a new List with the provided name and attributes configured via the List Config and returns the newly created list.
Definition
swat.createList(listConfig, onSuccess, onError)
Example
Here is an example demonstrating how to use this API to create a list named black friday list
:
// Define the list config
let listConfig = {
"lname": "black friday list", // unique list name.
};
// Define success callback
let onSuccess = function(newList) {
// Executed when a new list is successfully created
console.log("Successfully created a new List", newList);
}
// Define error callback
let onError = function(error) {
// Error is an xhrObject
console.log("Error while creating a List", error);
}
// Create a new list with the above callbacks and config
swat.createList(listConfig, onSuccess, onError);
List names must be unique.
If you attempt to create a new list using a name that already exists for another list, the create API will not generate a new list. Instead, it will return the existing list with the same name in the success response.
The shopper can now add products to the black friday list
. For adding a product to a list, please see Add to List API
API Parameters
Argument | Type | Description |
---|---|---|
listConfig | object | Please refer List Config |
onSuccess | function | Called when the List is successfully created. |
onError | function | Called when there is an error while creating the List. |
Success Response
The success response contains information about the duplicated List, like its name, type, lid
, created timestamp(cts
) , userInfo
and other useful metadata.
This information can be used to retrieve or manipulate the List in future API calls.
{
"di": "37ec0c4b-a930-4d13-87c1-ac08205402a1", // device id
"userinfo": null, // user info if logged in
"lty": "wl", // list type
"lprops": { // optional list properties.
"dry instructions": "tumble dry"
},
"cts": 1675665972622, // created timestamp
"lname": "black friday list", // list name
"lid": "59179e9f-139d-4857-bcdd-3ad88a38b47d", // list id
"pid": "KABSbuGyYCuM6/IV7e7cGxT1978LieU1jRdMt6XhYfo=", // unique merchant id
"uts": 1675665972622, // updated timestamp
"lhash": "YnVja2V0LWxpc3QtZm9yLXN1bW1lcg==" // list hash unique to list name
}
E.g, The shopper can now add a product to the list using the lid
from above created list.
The
lid
property is a crucial identifier for working with individual Lists.Each list is assigned a unique list ID ( lid). This ID enables you to pinpoint a specific list when using other API calls detailed in this documentation.
Duplicate an Existing List
The Create List API can be utilised to duplicate an existing list as well. To do so, include the lid
of the list you wish to duplicate as the value of the fromlid
property within the List Config object.
Here's an example of how you can use this API to duplicate a List:
Example
// Define the list config with the lid of the wishlist you want to duplicate
let listConfig = {
"lname": "My Duplicated List", // list name is required
"fromlid": "59179e9f-139d-4857-bcdd-3ad88a38b47d", // the lid of the List to duplicate
};
// Define success callback
let onSuccess = function(listObj) {
// Successfully duplicated a list
console.log("Successfully duplicated the existing List", listObj);
}
// Define error callback
let onError = function(error) {
// Error is an xhrObject
console.log("Error while duplicating a List", error);
}
// Duplicate a wishlist using the `createList` function
swat.createList(listConfig, onSuccess, onError);
Duplicate List names must be unique.
If you try to Duplicate a list with a name that is already in use by another list, the API will not Duplicate. It will return the existing list with the same name as part of the success response.
API Parameters
Argument | Type | Description |
---|---|---|
listConfig | object | Please refer List Config |
onSuccess | function | Called when the List is successfully duplicated. |
onError | function | Called when there is an error while duplicating the List. |
Success Response
The success response contains information about the duplicated List, like its name, type, lid
, created timestamp (cts
) , userInfo
and other useful metadata.
This information can be used to retrieve or manipulate the List in future API calls.
{
"di": "0fddb3d7-377d-48d0-b4eb-bc7f48b47478", // device ID
"lsrcname": "My SFL List", // name of the source list.
"lsrctype": "sfl", // type of the source list.
"userinfo": null, // information about the user (if logged in)
"lty": "wl", // type of the list (wishlist in this case)
"cts": 1678886977456, // creation timestamp
"lname": "New Wishlist 2", // name of the list
"lsrc": "ef3d664c-56a8-4ac2-b85e-3e2b48073e79", // ID of the source list (if any)
"lid": "5a8ce91c-7c84-475d-9d7a-eb9b885a2cef", // ID of the newly created list
"pid": "/IV7e7cGxT1978LieU1jRdMt6XhYfo=", // unique merchant ID
"uts": 1678886977456, // update timestamp
"lhash": "bmV3LXdpc2hsaXN0LTI=", // hash value of the list name
"_cp": 1 // copy
}
Other References
List Config
A simple Javascript object containing parameters required for creating a new List and duplicating an existing List
Property Name | Data Type | Required | Description |
---|---|---|---|
listConfig.lname | string | Yes | List name - unique for a given user, allows 3-50 characters. |
listConfig.lnote | string | optional | Note you may add to list, that you can fetch at a later time for frontend use |
listConfig.lprops | object | optional | Custom object that can store attrbiutes of your choice for each list. |
listConfig.fromlid | guid string | optional | Used to duplicate a list, when supplied this lid would be used to duplicate from |
listConfig.lty | string | optional | Specifies the type of list you want to create, wl - wishlist, sfl - save for later, this is used to create a different type of list separate from the wishlist |
Best Practices
Best Practices
- Keep the list name concise, clear, and unique for a given user.
- If duplicating a list, ensure that the new list name is different from the original list name.
- Use the
lprops
parameter to add custom fields or metadata to the list, if required.
Sample User Experience Flow
Here is a sample user experience seen in the wild. You can make your own user experience using the above APIs.
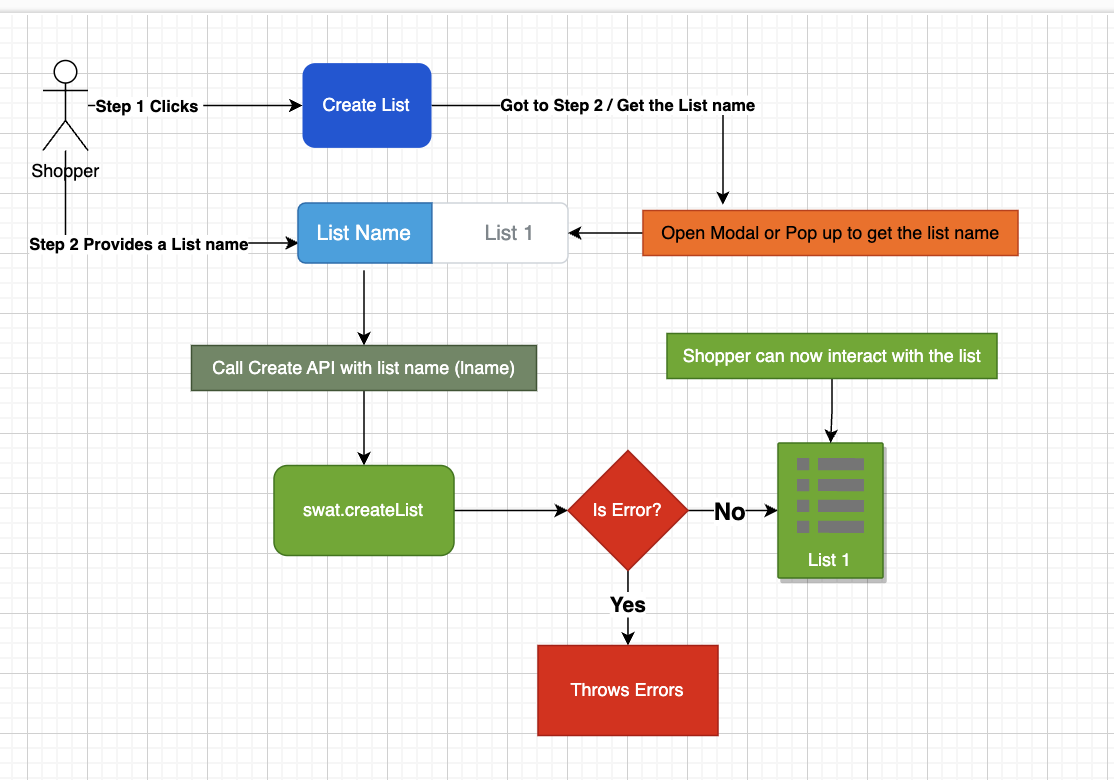
Sample Wishlist User Experience for Creating Lists.
Updated 10 months ago